老师,这个在数组中怎么测试时候出现不来结果呢?
来源:2-4 数组中查询元素和修改元素
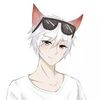
Clark_Chan
2019-02-27
package com.company;
public class Array {
private int[] data;
private int size;
//构造函数,传入构造函数的容量来创建数组Array
public Array(int capacity){
data=new int[capacity];
size=0;
}
public Array(){
this(10);
}
public int getSize(){
return size;
}
public int getCapacity(){
return data.length;
}
public boolean isEmpty(){
return size==0;
}
public void addLast(int e){
add(size,e);
}
public void addFirst(int e){
add(0,e);
}
public void add(int index,int e){
if(size==data.length){
throw new IllegalArgumentException("add is failed");
}
if (index<0||index>size){
throw new IllegalArgumentException("add is failed");
}
for (int i=size-1;i>=index;i--){
data[i+1]=data[i];
data[index]=e;
size++;
}
}
@Override
public String toString(){
StringBuilder res = new StringBuilder();
res.append(String.format("Array: size = %d , capacity = %d\n", size, data.length));
res.append('[');
for(int i = 0 ; i < size ; i ++){
res.append(data[i]);
if(i != size - 1)
res.append(", ");
}
res.append(']');
return res.toString();
}
}
package com.company;
public class Main {
public static void main(String[] args) {
Array arr = new Array(20);
for(int i = 0 ; i < 10 ; i ++){
arr.addLast(i);
}
System.out.println(arr);
}
}
运行这个方法出现的是这个结论,怎么不能像视频里按顺序出现0到9呢?
Array: size = 0 , capacity = 20
[]
写回答
2回答
-
Clark_Chan
提问者
2019-02-27
我在Main方法中直接循环然后打印 i的值是可以的,但是就arr.addLast(i),这个好像没添加进去值
20 -
liuyubobobo
2019-02-28
你的add代码是错误的。正确的代码如下:
// 在index索引的位置插入一个新元素e public void add(int index, int e){ if(size == data.length) throw new IllegalArgumentException("Add failed. Array is full."); if(index < 0 || index > size) throw new IllegalArgumentException("Add failed. Require index >= 0 and index <= size."); for(int i = size - 1; i >= index ; i --) data[i + 1] = data[i]; data[index] = e; size ++; }
注意,for循环中只有一句话。你将for循环外面的两句话也放到了for循环内。课程之前介绍过,对于只有一句的循环体或者if等语句,为了节省屏幕空间,我不使用大括号,而是用缩进表达。
请不要记忆for循环中应该有一句话还是三句话,一定要具体搞明白,为什么这样写,for循环到底在做什么事情。循环过程中,每一步,数据是如何变化的(结合课程中的动画演示)。
这个课程的所有代码都可以通过课程的官方github获得,遇到这种情况,也可以尝试在你的环境中运行课程的官方github,看是否有问题?如果没有问题,请仔细调试,对比,看自己的代码的问题在哪里。debug是学习算法非常重要的步骤哦:)
传送门:https://github.com/liuyubobobo/Play-with-Data-Structures
加油!:)
132019-10-08
相似问题