链表实现队列入栈的疑问
来源:4-7 带有尾指针的链表:使用链表实现队列
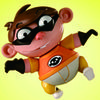
合之乎
2021-07-30
public void enqueue(E e){
if(tail == null){
tail = new Node(e);
head = tail;
}
else{
tail.next = new Node(e);
tail = tail.next;
}
size++;
}
实现添加队列的方法我有一些疑问:在多次调用该方法的过程中,只有第一次进行了tail是否为空的判断是成功的,因此运行了一次head = tail。但是之后的方法调用过程中没有调用head的痕迹,为什么head中Node对象依然可以不断变化。
以下是完整的代码:
public class LinkedListQueue<E> implements Queue<E>{
private class Node{
public E e;
public Node next;
public Node(E e, Node next){
this.e = e;
this.next = next;
}
public Node(E e){
this(e, null);
}
public Node(){
this(null, null);
}
@Override
public String toString(){
return e.toString();
}
}
private Node head, tail;
private int size;
public LinkedListQueue(){
head = null;
tail = null;
size = 0;
}
@Override
public int getSize() {
return size;
}
@Override
public boolean isEmpty() {
return size == 0;
}
@Override
public void enqueue(E e) {
if(tail == null){
tail = new Node(e);
head = tail;
}
else{
tail.next = new Node(e);
tail = tail.next;
}
size++;
}
@Override
public E dequeue() {
if(isEmpty())
throw new IllegalArgumentException("Cannot dequeue from an empty queue");
Node retNode = head;
head = head.next;
retNode.next = null;
if(head == null)
tail = null;
size--;
return retNode.e;
}
@Override
public E getFront() {
if(isEmpty())
throw new IllegalArgumentException("Queue is empty");
return head.e;
}
@Override
public String toString(){
StringBuilder res = new StringBuilder();
res.append("Queue: front ");
Node cur = head;
while(cur != null){
res.append(cur + "->");
cur = cur.next;
}
res.append("NULL tail");
return res.toString();
}
public static void main(String[] args) {
LinkedListQueue<Integer> queue = new LinkedListQueue<>();
for(int i = 0; i < 10; i++){
queue.enqueue(i);
System.out.println("---------------------------第" + (i+1) + "次循环---------------------------------" );
System.out.println("head对象的哈希值为:" + queue.head.hashCode());
System.out.println("tail对象的哈希值为:" + queue.tail.hashCode());
System.out.println("--------------------------------------------------------------------");
System.out.println(queue);
}
}
}
运行结果:
---------------------------第1次循环---------------------------------
head对象的哈希值为:586617651
tail对象的哈希值为:586617651
--------------------------------------------------------------------
Queue: front 0->NULL tail
---------------------------第2次循环---------------------------------
head对象的哈希值为:586617651
tail对象的哈希值为:998351292
--------------------------------------------------------------------
Queue: front 0->1->NULL tail
---------------------------第3次循环---------------------------------
head对象的哈希值为:586617651
tail对象的哈希值为:1684106402
--------------------------------------------------------------------
Queue: front 0->1->2->NULL tail
---------------------------第4次循环---------------------------------
head对象的哈希值为:586617651
tail对象的哈希值为:335471116
--------------------------------------------------------------------
Queue: front 0->1->2->3->NULL tail
---------------------------第5次循环---------------------------------
head对象的哈希值为:586617651
tail对象的哈希值为:1308927845
--------------------------------------------------------------------
Queue: front 0->1->2->3->4->NULL tail
---------------------------第6次循环---------------------------------
head对象的哈希值为:586617651
tail对象的哈希值为:2017354584
--------------------------------------------------------------------
Queue: front 0->1->2->3->4->5->NULL tail
---------------------------第7次循环---------------------------------
head对象的哈希值为:586617651
tail对象的哈希值为:391447681
--------------------------------------------------------------------
Queue: front 0->1->2->3->4->5->6->NULL tail
---------------------------第8次循环---------------------------------
head对象的哈希值为:586617651
tail对象的哈希值为:1935637221
--------------------------------------------------------------------
Queue: front 0->1->2->3->4->5->6->7->NULL tail
---------------------------第9次循环---------------------------------
head对象的哈希值为:586617651
tail对象的哈希值为:403424356
--------------------------------------------------------------------
Queue: front 0->1->2->3->4->5->6->7->8->NULL tail
---------------------------第10次循环---------------------------------
head对象的哈希值为:586617651
tail对象的哈希值为:321142942
--------------------------------------------------------------------
Queue: front 0->1->2->3->4->5->6->7->8->9->NULL tail
写回答
1回答
-
liuyubobobo
2021-07-31
如果单纯的调用 enqueue,head 在第一次设立以后,之后不会变化。请给我你的测试代码,说明结合你的测试代码,你为什么觉得 head 在变化?
P.S. 结合我的经验,请确认你的 toString 函数是正确的。
继续加油!:)
032021-07-31
相似问题