provider回的信息 searcher收不到
来源:3-7 案例实操-局域网搜索案例-3
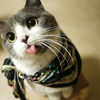
慕粉1890165
2021-01-23
package udp;
import java.io.IOException;
import java.net.DatagramPacket;
import java.net.DatagramSocket;
import java.util.UUID;
/**
-
UDP 提供者,用于提供服务
*/
public class UDPProvider {public static void main(String args[]) throws IOException {
String sn = UUID.randomUUID().toString(); Provider provider = new Provider(sn); provider.run(); //读取任意字符退出 System.in.read(); provider.exit();
}
private static class Provider extends Thread{
private String sn; private boolean done = false; private DatagramSocket ds = null; public Provider(String sn) { super(); this.sn = sn; } @Override public void run() { super.run(); System.out.println("UDPProvider started."); try{ ds = new DatagramSocket(20000); while(!done){ //构建接收实体 byte buf[] = new byte[512]; final DatagramPacket receivePacket = new DatagramPacket(buf,buf.length); //接收 ds.receive(receivePacket); //打印接收到的信息 String IP = receivePacket.getAddress().getHostAddress(); int PORT = receivePacket.getPort(); int length = receivePacket.getLength(); String data = new String(receivePacket.getData(),0,length); System.out.println("UDPProvider 接收到 ip: "+IP+"\tport:"+PORT+"\tLength"+length+"\tDATA"+data); int port = MessageCreator.parsePort(data); if(port!=-1){ String sendMessage = MessageCreator.buildWithPort(port); byte[] udpProviderSendByte = sendMessage.getBytes(); DatagramPacket sendGramPacket = new DatagramPacket(udpProviderSendByte,0,udpProviderSendByte.length, receivePacket.getAddress(),PORT); ds.send(sendGramPacket); } } }catch (Exception e){ }finally { exit(); } } public void exit(){ done = true; if(ds!=null){ System.out.println(ds.getInetAddress().getHostAddress()+"\t连接断开!"); ds.close(); ds = null; } }
}
}
package udp;
import java.net.DatagramPacket;
import java.net.DatagramSocket;
import java.net.InetAddress;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.CountDownLatch;
public class UDPSearcher {
private static final int LISTENPORT = 30000;
public static void main(String args[]) throws Exception {
Listener listener = listen();
sendBroadcast();
System.in.read();
List<Device> devices = listener.getDeviceAndClose();
for(Device device:devices ){
System.out.println(device.toString());
}
}
private static Listener listen() throws InterruptedException {
System.out.println("UDPSearcher start!");
CountDownLatch countDownLatch = new CountDownLatch(1);
Listener listener = new Listener(LISTENPORT,countDownLatch);
listener.start();
countDownLatch.await();
return listener;
}
private static class Device{
final int port;
final String ip;
final String sn;
private Device(int port, String ip, String sn) {
this.port = port;
this.ip = ip;
this.sn = sn;
}
@Override
public String toString() {
return "Device{" +
"port=" + port +
", ip='" + ip + '\'' +
", sn='" + sn + '\'' +
'}';
}
}
private static class Listener extends Thread {
private final int listenPort;
private final CountDownLatch countDownLatch;
private final List<Device> devices = new ArrayList<>();
private boolean done = false;
private DatagramSocket ds = null;
public Listener(int listenPort, CountDownLatch countDownLatch) {
this.listenPort = listenPort;
this.countDownLatch = countDownLatch;
}
@Override
public void run() {
super.run();
countDownLatch.countDown();
try{
ds = new DatagramSocket(listenPort);
while (!done){
byte buf[] = new byte[512];
final DatagramPacket receivePacket = new DatagramPacket(buf,buf.length);
//接收
ds.receive(receivePacket);
byte data[]= receivePacket.getData();
String receivesStr = new String(data,data.length);
String IP = receivePacket.getAddress().getHostAddress();
int PORT = receivePacket.getPort();
System.out.println("searcherSocket 接收到 ip: "+IP+"\tport:"+PORT+"\tLength"+data.length+"\tDATA"+receivesStr);
String SN = MessageCreator.parseSN(receivesStr);
if(SN!=null){
Device d = new Device(PORT,IP,SN);
devices.add(d);
}
}
System.out.println("UDPSearcher finish!");
}catch (Exception e){
}finally {
close();
}
}
private void close(){
done = true;
if(ds!=null){
ds.close();
ds = null;
}
}
private List<Device> getDeviceAndClose(){
close();
return devices;
}
}
public static void sendBroadcast() throws Exception {
System.out.println("UDPSearcher sendBroadcast started.");
DatagramSocket searcherSocket = new DatagramSocket();
String sendStr = MessageCreator.buildWithPort(LISTENPORT);
final DatagramPacket searcherPacket = new DatagramPacket(sendStr.getBytes(),sendStr.getBytes().length);
searcherPacket.setAddress(InetAddress.getByName("255.255.255.255"));
searcherPacket.setPort(20000);
searcherSocket.send(searcherPacket);
searcherSocket.close();
System.out.println("UDPSearcher sendBroadcast finish.");
}
}
写回答
1回答
-
Qiujuer
2021-01-25
问题应该是出在:MessageCreator
buildWithPort操作生成的字符串应该是无法给int port = MessageCreator.parsePort(data);进行解析。看逻辑是发送消息过去了,也收到了,但是没有回送消息;而没有回送消息应该是port解析失败了。
00
相似问题