如何获取Homd.js中state的数据
来源:5-4 价格题目列表测试分析和编写
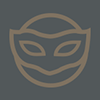
FanYiyang
2019-02-03
import React, { Component, Fragment } from 'react';
import PropTypes from 'prop-types';
import PriceList from '../../components/priceList';
import ViewTab from '../../components/viewTab';
import CreateBtn from '../../components/createBtn';
import TotalPrice from '../../components/totalPrice';
import MonthPicker from '../../components/monthPicker';
import { testCategories, testItems } from '../../config/testData';
import { LIST_VIEW, CHART_VIEW } from '../../config/action';
const newItem = {
'id': '_kly1klqw6',
'title': '新添加的项目',
'price': 300,
'date': '2019-02-01',
'cid': '12'
}
class Home extends Component {
state = {
arr: [],
totalIncome: 0,
totalOutcome: 0,
tabView: 'list',
currentDate: {}
}
componentWillMount () {
this.init()
}
// 初始化数据
init = (arrList = testItems) => {
const { currentDate } = this.state
const arr = arrList.map(item => {
testCategories.forEach(items => {
if (item.cid === items.id) {
item['category'] = items
}
})
return item
}).filter(item => {
return item.date.includes(`${currentDate.year}-0${currentDate.month}`)
})
console.log(arr)
const totalIncome = arr.filter(item => item.category.type === 'income').reduce((prev, item) => {
return item.price + prev
}, 0)
const totalOutcome = arr.filter(item => item.category.type === 'outcome').reduce((prev, item) => {
return item.price + prev
}, 0)
this.setState({
arr,
totalOutcome,
totalIncome
})
}
deleteItem = id => {
const arr = this.state.arr.filter(item => item.id !== id)
const totalIncome = arr.filter(item => item.category.type === 'income').reduce((prev, item) => {
return item.price + prev
}, 0)
const totalOutcome = arr.filter(item => item.category.type === 'outcome').reduce((prev, item) => {
return item.price + prev
}, 0)
this.setState({
arr,
totalOutcome,
totalIncome
})
}
onModifyItem = id => {
const arr = this.state.arr.map(item => {
if (item.id === id) {
return {...item, title: '更新后的标题'}
}else {
return item
}
})
this.setState({
arr
})
}
onTabChange = view => {
console.log(view)
this.setState({
tabView: view
})
}
changeDate = (year, month) => {
this.setState({
currentDate: { year, month }
}, () => {
this.init()
})
}
onCreateItem = () => {
const arr1 = [newItem, ...this.state.arr]
this.init(arr1)
}
render () {
const { arr, totalOutcome, totalIncome, tabView } = this.state
return (
<Fragment>
<header className='header-flex'>
<MonthPicker
changeDate={this.changeDate}/>
<TotalPrice
income={totalIncome}
outcome={totalOutcome}
/>
</header>
<main>
<ViewTab
onTabChange={this.onTabChange}
activeTab={tabView}
/>
<CreateBtn
onCreateItem={this.onCreateItem}
/>
<div className="content-area py-3 px-3">
{
tabView === LIST_VIEW &&
arr.map(item => {
return <PriceList
key={item.id}
iconName={item.category.iconName}
type={item.category.type}
title={item.title}
price={item.price}
date={item.date}
id={item.id}
onModifyItem={this.onModifyItem}
deleteItem={this.deleteItem}
/>
})
}
{
tabView === CHART_VIEW &&
<h1>这就是我的</h1>
}
</div>
</main>
</Fragment>
)
}
}
export default Home;
这是测试文件
import React from 'react';
import { shallow, mount } from 'enzyme';
import PriceList from '../priceList';
import { testCategories, testItems } from '../../config/testData';
import { arr } from '../../containers/home';
const props = {
onModifyItem: () => {},
deleteItem: () => {},
arr,
}
jest.mock('../../config/testData');
console.log(arr)
let wrapper
describe('test PriceList component', () => {
// 每次都会执行
beforeEach(() => {
wrapper = shallow(<PriceList {...props} />)
// wrapper = mount(<PriceList />)
})
afterEach(() => {
wrapper.unmount();
});
it('should render the component to match snapshot', () => {
expect(wrapper).toMatchSnapshot()
})
})
我应该如何在测试文件中获取到home组件中arr的数据
写回答
1回答
-
张轩
2019-02-11
同学 针对你问题之外,我想谈谈你代码里面的问题,我们都说过 state 状态应该是数据的最小化合集,totalIncome 和 totalOutcome 完全就没有必要放到 state 当中,因为他们完全在每次 render 的时候通过 arr 计算出来,而且 this.init 方法也不需要 在 componentWillMount 中调用,你可以在 constructor 的时候直接 把 testItems 赋值给 this.state.arr.
好,现在回到你的问题,如果你想测试一个 组件的 state,你可以使用 wrapper.state() 它返回的是一个对象,包含你所有的 state。 https://airbnb.io/enzyme/docs/api/ReactWrapper/state.html
00
相似问题