关于leetcode126题,第32个用例过不去,目前的代码还有优化空间吗?(第二版)
来源:7-6 Leetcode 上一个困难的问题
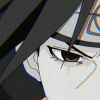
Llizzzc
2024-03-27
import java.util.*;
public class Solution2 {
private List<List<String>> res = new ArrayList<>();
private HashSet<Integer>[] G;
private List<String> wordList;
private int[] visitedBFS;
private boolean[] visitedDFS;
private String beginWord;
private String endWord;
private int shortest;
public List<List<String>> findLadders(String beginWord, String endWord, List<String> wordList) {
if (!wordList.contains(endWord)) {
return res;
}
if (!wordList.contains(beginWord)) {
wordList.add(beginWord);
}
this.wordList = wordList;
this.beginWord = beginWord;
this.endWord = endWord;
visitedBFS = new int[wordList.size()];
Arrays.fill(visitedBFS, -1);
visitedDFS = new boolean[wordList.size()];
int endIndex = 0;
for (; endIndex < wordList.size(); endIndex ++) {
if (wordList.get(endIndex).equals(endWord)) {
break;
}
}
G = new HashSet[wordList.size()];
constructGraph();
bfs(endIndex);
List<String> path = new ArrayList<>();
path.add(wordList.get(endIndex));
dfs(endIndex, path, 1);
return res;
}
// 建图
private void constructGraph() {
for (int i = 0; i < G.length; i ++) {
G[i] = new HashSet<>();
}
for (int i = 0; i < wordList.size(); i ++) {
for (int j = i + 1; j < wordList.size(); j ++) {
if (isConnected(i, j)) {
G[i].add(j);
G[j].add(i);
}
}
}
}
// 判断是否相邻
private boolean isConnected(int i, int j) {
String s1 = wordList.get(i);
String s2 = wordList.get(j);
int count = 0;
for (int k = 0; k < s1.length(); k ++) {
if (s1.charAt(k) != s2.charAt(k)) {
count ++;
}
if (count > 1) {
return false;
}
}
return true;
}
// 查找最短路径长度
private void bfs(int endIndex) {
Queue<Integer> queue = new ArrayDeque<>();
queue.add(endIndex);
visitedBFS[endIndex] = 1;
while (!queue.isEmpty()) {
int cur = queue.remove();
for (int next : G[cur]) {
if (visitedBFS[next] == -1) {
visitedBFS[next] = visitedBFS[cur] + 1;
queue.add(next);
if (wordList.get(next).equals(beginWord)) {
shortest = visitedBFS[next];
return;
}
}
}
}
}
private void dfs(int cur, List<String> path, int count) {
if (count > shortest) {
return;
}
if (count == shortest) {
if (wordList.get(cur).equals(beginWord)) {
res.add(new ArrayList<>(path));
}
return;
}
for (int next : G[cur]) {
if (!visitedDFS[next]) {
visitedDFS[next] = true;
path.addFirst(wordList.get(next));
dfs(next, path, count + 1);
path.removeFirst();
visitedDFS[next] = false;
}
}
}
}
写回答
1回答
-
liuyubobobo
2024-03-28
status = findStatus(cur);
这步比较慢。比较慢的原因其实更多在于字符串的处理速度。在这过程中,你要生成 500 * 5 * 26 (cur 的可能数 * cur 的长度 * 每个位置的字母可能数) 这么多新的字符串,然后去判断这些字符串在不在一个哈希表中。判断一个字符串是否在哈希表中也远比判断一个数字是否在哈希表中慢。
一个更快的做法是:基于初始的 wordList(和 beginWord),给每一个 word 编号,然后直接基于这个编号先把图给建出来。建这个图的过程,一个双重循环比较每两个 word 是否相邻。比较的过程中不需要创建新的字符串(这是非常重要的提速,new 的内存开销是很大的。)
这样,你可以直接基于这个 int 类型的图做 bfs,在这个图中,判断一个点是否访问过也不需要字符串的哈希表,直接使用数组就可以(visitedBFS 可以是数组,这也是一个重要提速。虽然理论哈希表的提取速度是 O(1),但实际远远慢于数组,甚至慢 10 倍都是正常的。)visitedDFS 同理可以是数组。
核心是,在算法的核心,都在操作 int 型,只是在初始的时候,建图的过程,把 string 映射成了 int。在最后获得答案的时候,再把 int 转换成了对应的 string。
你可以先使用这个思路修改一下试试看。如果还超时,我可以再给你写一个参考代码。
继续加油!:)
022024-03-29
相似问题