一直显示非法来源 但是网址是正常的
来源:9-12 登录验证客户用户表视频表创建外链视频功能开发-下(2)
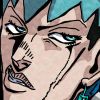
LOCALHOST90990
2021-07-31
views/Video.py from django.views.generic import View from app.libs.base_render import render_to_response from django.shortcuts import redirect, reverse from django.contrib.auth import login, logout, authenticate from django.contrib.auth.models import User from django.core.paginator import Paginator from app.utils.permission import dashboard_auth from app.model.video import VideoType, FromType, NationalityType, Video from app.utils.common import * class ExternaVideo(View): TEMPLATE = 'dashboard/video/externa_video.html' @dashboard_auth def get(self, request): error = request.GET.get('error', '') data = {'error': error} return render_to_response(request, self.TEMPLATE, data) def post(self, request): name = request.POST.get('name') image = request.POST.get('image') video_type = request.POST.get('video_type') from_to = request.POST.get('from_to') nationality = request.POST.get('nationality') info = request .POST.get('info') #print(name, image, video_type, from_to, nationality) if not all([name, image, video_type, from_to, nationality, info]): return redirect('{}?error={}'.format(reverse('externa_video'), '缺少必要字段')) result = check_and_get_video_type(VideoType, video_type, '非法的视频类型') if result.get('code') != 0: return redirect('{}?error={}'.format(reverse('externa_video'), result['msg'])) result = check_and_get_video_type(FromType, from_to, '非法来源') if result.get('code') != 0: return redirect('{}?error={}'.format(reverse('externa_video'), result['msg'])) result = check_and_get_video_type(NationalityType, nationality, '非法的国籍信息') if result.get('code') != 0: return redirect('{}?error={}'.format(reverse('externa_video'), result['msg'])) Video.objects.create( name=name, image=image, video_type=video_type, from_to=from_to, nationality=nationality, info=info ) return redirect(reverse('externa_video'))
common.py
# coding :utf-8 def check_and_get_video_type(type_obj, type_value, message): try: type_obj(type_value) except: return {'code': -1, 'msg': message} return {'code': 0, 'msg': 'success'}
externa_video.html:
<%inherit file="../base.html" /> <%! from django.shortcuts import reverse %> <%def name="content()"> <% from app.model.video import VideoType, FromType, NationalityType %> <h1>外链视频</h1> <button class="btn btn-info open-btn " id="open-add-video-btn">创建</button><span>${error}</span> <form id="video-edit-area" class="form-horizontal edit-area add-video-area" action="" method="POST">${csrf_token} <div class="form-group"> <div class="col-sm-10"> <input type="text" class="form-control" name="name" placeholder="视频名称"> </div> </div> <div class="form-group"> <div class="col-sm-10"> <input type="text" class="form-control" name="image" placeholder="海报"> </div> </div> <div class="form-group"> <div class="col-sm-10"> <label>视频类型</label> <select class="form-control video-select" name="video_type"> %for video_type in VideoType: <option value="${video_type.value}">${video_type.label}</option> %endfor </select> </div> </div> <div class="form-group"> <div class="col-sm-10"> <label>来源</label> <select class="form-control video-select" name="from_to"> %for from_to in FromType: %if from_to != FromType('custom'): <option value="${video_type.value}">${from_to.label}</option> %endif %endfor </select> </div> </div> <div class="form-group"> <div class="col-sm-10"> <label>国籍</label> <select class="form-control video-select" name="nationality"> %for nationality in NationalityType: <option value="${nationality.value}">${nationality.label}</option> %endfor </select> </div> </div> <div class="form-group"> <div class="col-sm-10"> <textarea name="info" class="form-control" placeholder="简介"></textarea> </div> </div> <button type="submit" class="btn btn-info">添加</button> </form> </%def> <%def name="css()"> <link href="/static/dashboard/css/externa_video.css" rel="stylesheet" /> </%def> <%def name="js()"> <script src="/static/dashboard/js/externa_video.js"></script> </%def>
效果:
model/video.py
from enum import Enum from django.db import models class VideoType(Enum): movie = 'movie' cartoon = 'cartoon' episode = 'episode' variety = 'variety' other = 'other' VideoType.movie.label = '电影' VideoType.cartoon.label = '动漫' VideoType.episode.label = '电视剧' VideoType.variety.label = '综艺' VideoType.other.label = '其它' class FromType(Enum): youku = 'youku' aiqiyi = 'aiqiyi' bilibili = 'bilibili' custom = 'custom' FromType.youku.label = '优酷' FromType.aiqiyi.label = '爱奇艺' FromType.bilibili.label = '哔哩哔哩' FromType.custom.label = '自制' class NationalityType(Enum): china = 'china' japan = 'japan' korea = 'korea' america = 'america' other = 'other' NationalityType.china.label = '中国' NationalityType.japan.label = '日本' NationalityType.korea.label = '韩国' NationalityType.america.label = '美国' NationalityType.other.label = '其它' class Video(models.Model): name = models.CharField(max_length=100, null=False) image = models.CharField(max_length=500, default='') video_type = models.CharField(max_length=50, default=VideoType.other.value) from_to = models.CharField(max_length=20, null=False, default=FromType.custom.value) nationality = models.CharField(max_length=20, default=NationalityType.other.value) info = models.TextField() status = models.BooleanField(default=True, db_index=True) created_time = models.DateTimeField(auto_now_add=True) update_time = models.DateTimeField(auto_now=True) # 联合唯一索引 如 防止重名 如 变形金刚 class Meta: unique_together = ('name', 'video_type', 'from_to', 'nationality') def __str__(self): return self.name class VideoStar(models.Model): #外键关联 video = models.ForeignKey(Video, related_name='video_star', on_delete=models.SET_NULL, blank=True, null=True) name = models.CharField(max_length=100, null=False) identity = models.CharField(max_length=50, default='') class Meta: unique_together = ('video', 'name', 'identity') def __str__(self): return self.name class VideoSub(models.Model): video = models.ForeignKey( Video, related_name='video_sub', on_delete=models.SET_NULL, blank=True, null=True ) url = models.CharField(max_length=500, null=False) number = models.IntegerField(default=1) class Meta: unique_together = ('video', 'number') def __str__(self): return 'video:{}, number:{}'.format(self.video.name, self.video.number) #number
写回答
1回答
-
deweizhang
2021-08-01
用的是豆瓣那个联系吗?那个联系制作为了解,不用做实际课程。咱们视频里有说明,因为豆瓣接口后来改了 禁止访问了
00
相似问题