怎么防止抓包
来源:1-2 课程导学
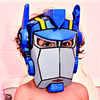
慕设计5218244
2024-05-27
怎么防止Flutter应用被抓包,例如防止被Charles和HttpCanary抓包?
写回答
1回答
-
CrazyCodeBoy
2024-05-28
为了防止Flutter应用被抓包,你可以采取以下一些安全措施。这些措施可以减少应用程序在网络传输过程中被拦截和分析的风险:
### 1. 使用HTTPS
始终使用HTTPS(而不是HTTP)进行所有的网络通信。HTTPS加密通信内容,防止被简单地读取和篡改。
### 2. 使用证书固定(Certificate Pinning)
证书固定可以确保应用只接受特定证书或公钥,从而防止中间人攻击。你可以通过在应用中嵌入可信的证书信息来实现证书固定。
以下是如何在Flutter应用中实现证书固定:
#### 依赖库
使用`http`库和`http_certificate_pinning`库进行证书固定:
```yaml
dependencies:
flutter:
sdk: flutter
http: ^0.13.3
http_certificate_pinning: ^2.1.0
```
#### 示例代码
```dart
import 'package:flutter/material.dart';
import 'package:http/http.dart' as http;
import 'package:http_certificate_pinning/http_certificate_pinning.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text('Certificate Pinning Example')),
body: Center(
child: ElevatedButton(
onPressed: _makeSecureRequest,
child: Text('Make Secure Request'),
),
),
),
);
}
Future<void> _makeSecureRequest() async {
var url = 'https://your-secure-api.com/endpoint';
var result = await HttpCertificatePinning.check(
serverURL: url,
headerHttp: {},
sha: SHA.SHA256,
allowedSHAFingerprints: ["your_cert_sha256_fingerprint"],
timeout: 50,
);
if (result == VerifyResult.success) {
// Make your request using the http package
var response = await http.get(Uri.parse(url));
print('Response: ${response.body}');
} else {
print('Certificate pinning verification failed.');
}
}
}
```
#### 解释:
- `allowedSHAFingerprints`:替换为你的证书的SHA256指纹。
- `HttpCertificatePinning.check`:用来验证服务器证书的指纹是否匹配。
### 3. 网络请求加密
在数据敏感的情况下,可以对数据进行额外的加密,然后在服务器端进行解密。这样即使传输层被拦截,数据内容也不会被直接读取。
### 4. 使用SSL/TLS Pinning
这种方法类似于证书固定,但可以在代码中更灵活地实现。通过自定义的HTTP客户端,检查服务器的SSL/TLS证书。
### 5. 混淆代码
使用代码混淆来增加逆向工程的难度。在发布应用时启用混淆(ProGuard/R8),隐藏敏感代码逻辑。
#### 示例(Android ProGuard):
在`android/app/build.gradle`中启用ProGuard:
```gradle
android {
buildTypes {
release {
minifyEnabled true
useProguard true
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
}
```
### 6. 安全提示
- **不要在代码中硬编码敏感信息**:如API密钥、密码等。
- **使用安全的凭证存储**:如Keychain(iOS)和Keystore(Android)。
- **定期更新和监控**:保持依赖库和应用的最新版本,及时修复已知的安全漏洞。
### 7. 检测调试和模拟环境
检测应用是否在调试模式下运行或是否在模拟器中运行,并根据需要限制或禁用某些功能。
#### 示例代码:
```dart
import 'dart:io';
bool isInDebugMode = false;
void main() {
isInDebugMode = _isDebugMode();
runApp(MyApp());
}
bool _isDebugMode() {
bool inDebugMode = false;
assert(inDebugMode = true);
return inDebugMode;
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
if (isInDebugMode) {
print("Running in debug mode. Certain features are disabled.");
}
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text('Anti-Debug Example')),
body: Center(
child: Text(isInDebugMode ? 'Debug Mode' : 'Release Mode'),
),
),
);
}
}
```
通过这些措施,可以显著提高Flutter应用的安全性,防止被抓包和其他中间人攻击。在生产环境中,确保全面考虑和实施这些安全策略,保护用户的数据和隐私。00
相似问题