9、查看班级平均成绩,请彭彭老师检查,感谢!
来源:14-17 测评作业
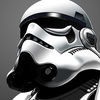
mottoyin
2025-02-20
ClassScore类
package com.imooc.chapter14test;
public class ClassScore {
private float chineseScore; //语文平均分
private float mathScore; //数学平均分
private float englishScore; //英语平均分
//创建无参构造方法
public ClassScore() {
}
//创建含参构造方法
public ClassScore(float chineseScore, float mathScore, float englishScore) {
this.chineseScore = chineseScore;
this.mathScore = mathScore;
this.englishScore = englishScore;
}
public float getChineseScore() {
return chineseScore;
}
public void setChineseScore(float chineseScore) {
this.chineseScore = chineseScore;
}
public float getMathScore() {
return mathScore;
}
public void setMathScore(float mathScore) {
this.mathScore = mathScore;
}
public float getEnglishScore() {
return englishScore;
}
public void setEnglishScore(float englishScore) {
this.englishScore = englishScore;
}
//重写toString方法
@Override
public String toString() {
return "语文: " + chineseScore +
",数学: " + mathScore +
",英语: " + englishScore
;
}
}
测试类
package com.imooc.chapter14test;
import java.util.*;
public class ClassScoreTest {
public static void main(String[] args) {
//初始化5个ClassScore对象
ClassScore cs1 = new ClassScore(96.5f,98.8f,95.2f);
ClassScore cs2 = new ClassScore(96.7f,97.5f,96.5f);
ClassScore cs3 = new ClassScore(95.9f,98.2f,95.5f);
ClassScore cs4 = new ClassScore(97.3f,97.9f,96.8f);
ClassScore cs5 = new ClassScore(96.6f,98.5f,96.3f);
//创建一年级hashMap集合
Map<String, ClassScore> classMap = new HashMap<>();
classMap.put("一年1班",cs1);
classMap.put("一年2班",cs2);
classMap.put("一年3班",cs3);
classMap.put("一年4班",cs4);
classMap.put("一年5班",cs5);
//使用Entry对象遍历Map总的元素
Set<Map.Entry<String, ClassScore>> entrySet = classMap.entrySet();
for (Map.Entry<String, ClassScore> item:
entrySet) {
System.out.println(item.getKey() + "-> " + item.getValue());
}
}
}
写回答
1回答
-
彭彭老师
2025-02-20
没有问题,完成的很好
00
相似问题