TypeError: Expected binary or unicode string, got Dimension(8)
来源:3-6 使子类与lambda分别实战自定义层次
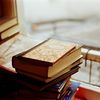
慕云19
2019-09-06
import matplotlib as mpl import matplotlib.pyplot as plt import numpy as np import sklearn import pandas as pd import os import sys import tensorflow as tf from tensorflow import keras from sklearn.preprocessing import StandardScaler from sklearn.datasets import fetch_california_housing import pprint from sklearn.model_selection import train_test_split from sklearn.preprocessing import StandardScaler print(sys.version_info) for module in mpl,np,pd,sklearn,tf,keras: print(module.__name__,module.__version__) housing = fetch_california_housing() print(housing.DESCR) print(housing.data) print(housing.data.shape) print(housing.target) print(housing.target.shape) pprint.pprint(housing.data) pprint.pprint(housing.target) print(housing.data[10]) x_train_all,x_test,y_train_all,y_test = train_test_split(housing.data,housing.target,random_state=7,test_size=0.25) x_train,x_valid,y_train,y_valid = train_test_split(x_train_all,y_train_all,random_state=11,test_size=0.25) print(x_train.shape,y_train.shape) print(x_valid.shape,y_valid.shape) print(x_test.shape,y_test.shape) scaler = StandardScaler() x_train_scaled = scaler.fit_transform(x_train) x_valid_scaled = scaler.transform(x_valid) x_test_scaled = scaler.transform(x_test) def customized_mse(y_true,y_pre): return tf.reduce_mean(tf.square(y_pre - y_true)) # customized dense layer class CustomizedDenseLayer(keras.layers.Layer): def __init__(self,units,activation=None,**kwargs): self.units = units self.activation = keras.layers.Activation(activation) super(CustomizedDenseLayer,self).__init__(**kwargs) def build(self, input_shape): '''构建所需要的参数''' # x * w + b input_shape:[None,a] w:[a,b] output_shape:[None,b] #add_weight获得一个变量 self.kernel = self.add_weight(name='kernel', shape=(input_shape[1],self.units), initializer='uniform', #定义如何初始化参数矩阵,uniform:均匀分布的方法 trainable=True) self.bias = self.add_weight(name='bias', shape=(self.units), initializer='zeros', trainable=True) super(CustomizedDenseLayer,self).build(input_shape) def call(self, x): '''完成正向计算''' return self.activation(tf.matmul(x,self.kernel) + self.bias) model = keras.models.Sequential([ CustomizedDenseLayer(30,activation='relu', input_shape=x_train.shape[1:]), CustomizedDenseLayer(1) ]) model.summary() sgd = keras.optimizers.SGD(lr=0.001) model.compile(loss=customized_mse,optimizer=sgd,metrics=['mean_squared_error']) callbacks = [keras.callbacks.EarlyStopping(patience=5,min_delta=1e-2,monitor='val_loss')] history = model.fit(x_train_scaled,y_train, validation_data=(x_valid_scaled,y_valid), epochs=100, callbacks=callbacks) model.evaluate(x_test_scaled,y_test) def plot_learning_curves(history): pd.DataFrame(history.history).plot(figsize=(8,5)) plt.grid(True) plt.gca().set_ylim(0,1) plt.show() plot_learning_curves(history)
老师,请问为什么会报错:TypeError: Failed to convert object of type <class 'tuple'> to Tensor. Contents: (Dimension(8), 30). Consider casting elements to a supported type.
写回答
1回答
-
正十七
2019-10-15
同学你好,我运行了你的代码,没有问题啊。你的tensorflow的版本是什么?是不是版本的问题?
00
相似问题